Updated
Published
Using dot env files with Node.js
Manage your environment variables with Node.js
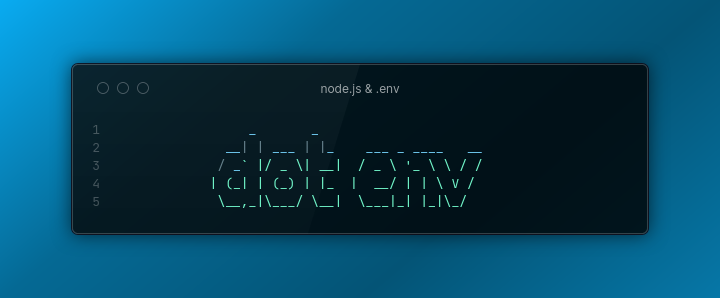
Environment variables, or env vars for short, are commonly used to store configuration and secrets for use with a program. Separating configuration lets programmers avoid hardcoding values like database credentials into a program’s code. Having them separate also helps avoid publishing secrets to repositories.
Table of contents
- How to use Environment Variables in Node.js
- Storing Environment Variables in .env Files
- Loading .env Files in Node.js 20.6 and Above
- Loading .env Files in Node.js 20.5 and Below
- Other Tools & Resources
How to use Environment Variables in Node.js
With Node.js you can easily access env vars by using
process.env
.
For example, this script will print whatever is in the DB_PASSWORD
variable:
console.log(process.env.DB_PASSWORD);
You can run this script in a variety of different ways:
$ node example.js
undefined
$ DB_PASSWORD=foo node example.js
food
$ export DB_PASSWORD=bar
$ node example.js
bar
As shown in the above example, if not set the value in process.env
will be undefined.
Many times it will be useful to set a default as with the below example:
const password = process.env.DB_PASSWORD || 'password';
console.log(password);
Storing Environment Variables in .env Files
Rather than having to specify each environment variable on the command line before
running a script, env vars can be placed in a .env
file. This can be very helpful
when dealing with a large number of variables.
.env
files are generally specified in the “ini” format which looks like this:
API_KEY=abcd
# Database config
DB_PASSWORD=foo
DB_USER=root
As of Node.js v21.7.0, multi-line values are now supported.
MULTI_LINE="HELLO
WORLD"
Loading .env Files in Node.js 20.6 and Above
As of Node.js version 20.6.0, Node
will load a .env
file for you. Pass the --env-file
to node
along with a .env
file and the contents of the file will be loaded
into process.env
.
$ node --env-file=.env example.js
Alternatively, you can load .env
files using process.loadEnvFile('/path/to/.env')
(From Node.js v21.7.0 onward).
This function
will load the specified .env
file into process.env
without overwriting existing
variables. If the function is called without a path specified, it will use the .env
file in the current directory. It is important to note that it will not look
in parent directories to find a .env
file, it will throw an error if a .env
file does not exist in the current directory.
Loading .env Files in Node.js 20.5 and Below
Prior to Node.js version 20.6.0, you need to use an NPM package to load .env
files
into process.env
(or roll your own!). One of the more popular packages is
dotenv
, which can be installed by running
npm install dotenv
. Then you just need to import the dotenv
package and it will
automatically find and parse your .env
file. For example:
require('dotenv').config();
console.log(process.env.DB_PASSWORD);
Other Tools & Resources
When working on a large project with many team members and/or many environment variables it may become necessary to use a configuration and secrets manager. The one currently being used at my job is EnvKey. EnvKey makes it simple to manage credentials and configurations for multiple different environments and manage access to those credentials. There are other options out there, EnvKey just happens to be the tool that I am most familiar with.